Since .NET 9, Blazor makes it easy to manage cache problems for our static resources (CSS and JS). Prior to this update, I had written an article on how to get the best out of cache-busting(https://www.peug.net/blazor-astuce-de-cache-busting-pour-mes-fichiers-css/ ) Lucky you can migrate to .NET 9 straight away! Let’s have a look:
What will we end up with?
The result is that Blazor will modify the name of our static files by adding a small hash which will be a fingerprint made with the file. This hash will only change if the file is modified. We will therefore have :
Set up
There’s not much to do: in program.cs, replace app.UseStaticFiles(), which remains compatible, with app.MapStaticAssets(). MapStaticAsset will compress static resources with gzip during the application generation process and with brotli during publication (to further reduce download size). In addition, and this is what we’re interested in, it will generate thefingerprints of our static resources when the application is published.
Next, we’ll simply tag the path to our style sheet with @Asset[]:
About compression brotli, on my application, for example that for my CSS I have a gain of 46% :
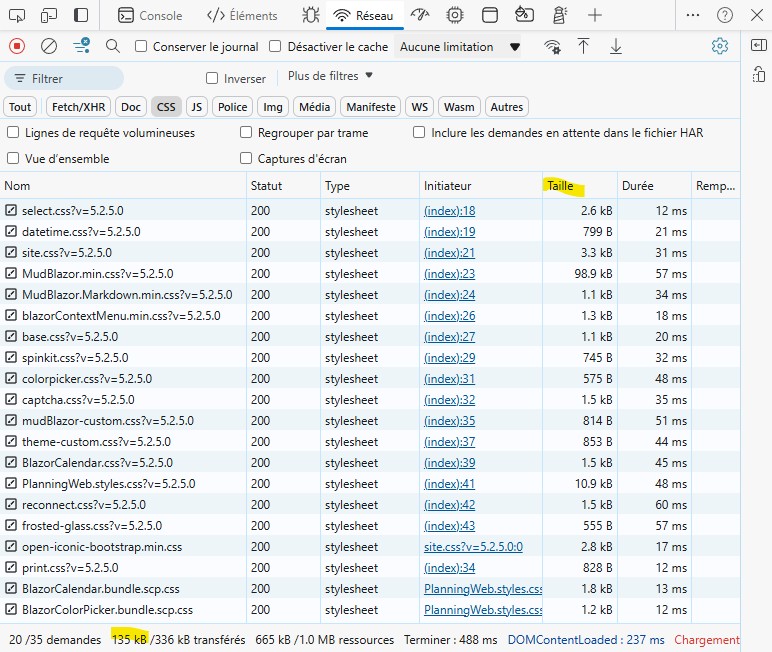
Et ensuite avec dotnet 9 :
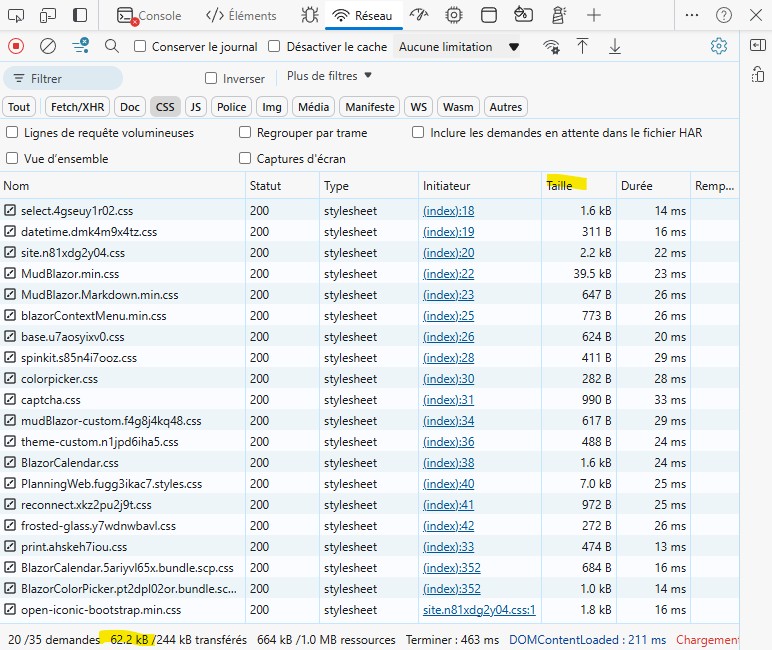
But that’s not all, let’s have a look at the rest:
About Javascript files
JS files are part of our static resources and should therefore be managed in the same way, even if you have placed them at the bottom of your <body> tag.
However, there will be a small peculiarity concerning Javascript modules, which will become more and more widespread as time goes by. Since ES6 (2015) it has been easier to write JS code in independent files. Each module can export elements (functions, objects, classes, etc.) that other files can import according to their needs. This encourages better organisation, limits global variable conflicts and makes code maintenance easier. And with Blazor they are loaded only when they are needed and unloaded at the end.
Example of this small module:
That’s how I use it with Blazor:
The problem we had before .NET9 was that when debugging JS code, we had to add a version parameter to make sure we were executing the right code: ‘/js/datetime.js?v1’. Anyway, that was before.
For JS module caching, all you have to do is add <ImportMap /> to the <head> of your app and that’s it. This is a fairly new trick ^^ It will create an import map of all the JS modules(https://developer.mozilla.org/fr/docs/Web/HTML/Element/script/type/importmap )
So when you run your code, the framework will take care of referencing all your JS modules in your code to create the following script, which will replace the previously added base:
Remarks
- Warning: if you still have static files with a version parameter (e.g. < link rel=‘stylesheet “ href= ”@Assets[’ css/datetime.css?v1’ ] ’ ) this will not work.
- If the path to your static file is misspelled (a slash at the beginning) (e.g. ‘ /css/datetime.css’), it won’t work.
- Has no effect on the static files of the Nugets packages you use ( <link href=‘@Assets[’_content/Microsoft.FluentUI.AspNetCore.Components/css/reboot.css‘] “ rel=”stylesheet’ /> : this won’t work. You might as well wait for this package to implement it in a future version.